I'm trying to utilise APIs that upload files to existing studio projects which are published to Groupshare; however, I'm not sure how to locate the source folder where I should put the files in.
Here are the APIs I'm trying to use:
POST /api/projectserver/v2/projects/{projectId}/files/upload
PUT /api/projectserver/v2/projects/{projectId}/files/{languageFileId}/upload
POST /api/projectserver/v4/projects/{projectGuid}/update
POST /api/projectserver/v4/projects/{projectId}/files/upload/perfectmatch
Please note that I'm trying to use my machine not the console's environment.
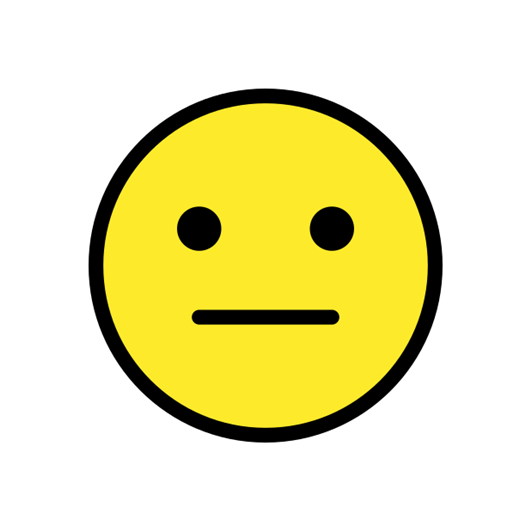